Earlier this week I set out to convert a few of my Angular 2 apps to use Ahead-of-time (AoT) compilation. I’ve just been to AngularConnect and heard about a project named “@ngtools/webpack” (video here) which supposedly allows you to use the same build tools that the angular-cli project uses, even if you don’t use the cli (which is the case with my stuff right now).
So I eagerly searched out@ngtools/webpack on npm and found this:
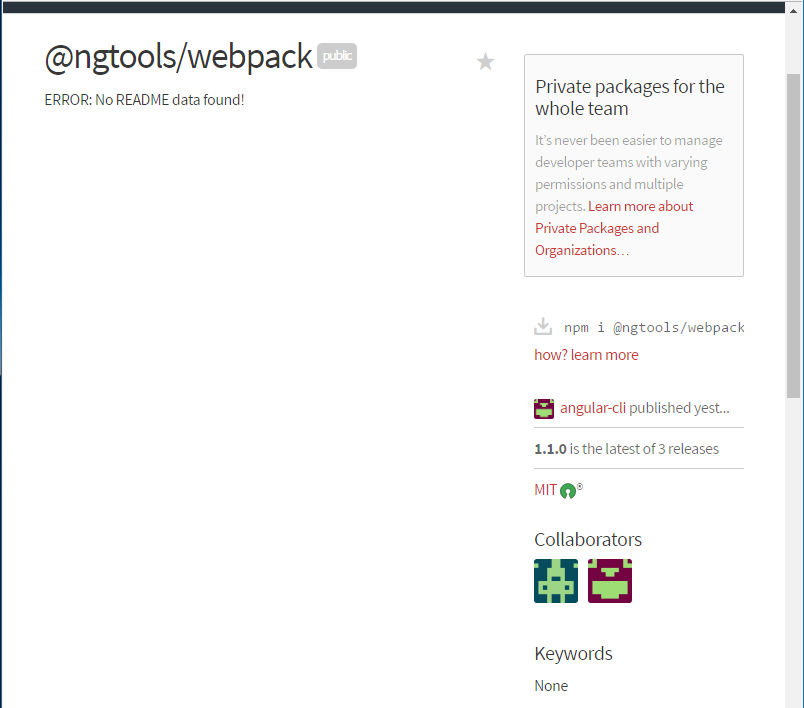
The @ngtools/webpack npm listing at the moment
No readme, no repo, nothing.
So I took a few (dark, frustrating) hours sniffing around and eventually got my apps working. When I mentioned this on Twitter there seemed to be a lot of interest, so I thought I’d quickly note down the things I found that helped me to implement AoT in my apps.
Note: This is not an exhaustive tutorial. I fully expect the project authors to document all this at some point, and they will do a much better job than I could. So these are just some notes to point you in the right direction. You’ll have to roll your sleeves up too, okay?
What You Need to Know
Here’s what I found which lead me to figure it all out. Note: I’m on Angular 2.0.2 at this time.
- If you’ve not already, read the angular.io docs on AoT. This will tell you the basic requirements and concepts.
- The @ngtools/webpack project does not have its own repo. It is located here in the angular-cli repo. The readme is here.
- The key to putting it all together was this example app, also hidden deep within the angular-cli repo. Study it carefully. Between the webpack.config.js, package.json & tsconfig.json, it actually contains everything you need to know.
- You need to update to TypeScript 2.
- You need to update to Webpack 2. This will probably involve a few changes to your webpack.config.js file, but Webpack 2 is actually very good at instructing you on what is broken. Just read the advice in the command line.
- You cannot use
require('..')
to load your templates or styles in AoT mode. You’ll need to convert totemplateUrl
andstyleUrls
. Don’t worry though, the @ngtools/webpack plugin will handle the Sass files automatically. For things to keep working when developing in JiT mode, you’ll need angular2-template-loader. - I had some trouble with the path to the ngfactory code when compiling with ngc:
Can't resolve './../path/to/app/app.module.ngfactory'
This seems related to this issue. The solution for me was to define thegenDir
in the webpack config rather than the tsconfig.js file.
My ng2-pagination project now includes a demo app which is set up to run in JiT mode for dev, and AoT mode for distribution. Here is the webpack config file.
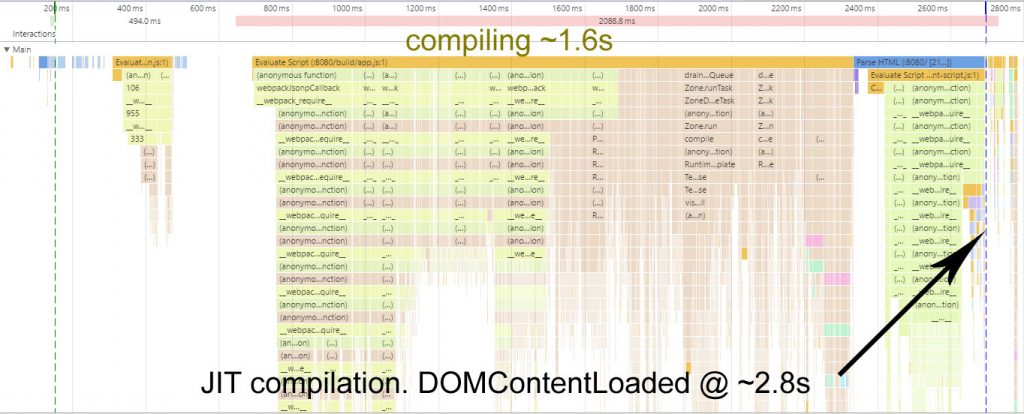
Chrome timeline for ng2-pagination demo app, JiT mode.
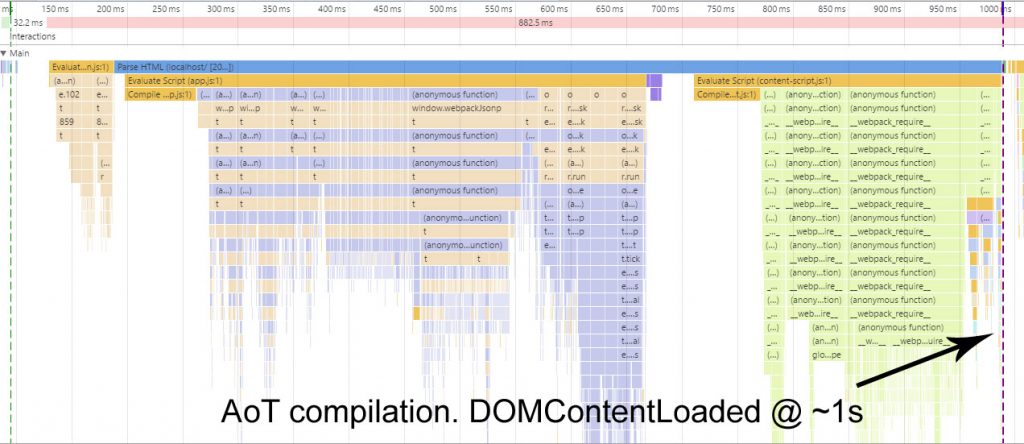
Chrome timeline for ng2-pagination demo app, AoT mode.
Disclaimer
Even knowing the above, you will run into snags and issues along the way. Just hopefully fewer than I did. Maybe some of the above is incorrect; without official documentation, it can be difficult to know. Seems to work so far though. I expect that in the coming weeks, this article will become obsolete.
Sharpen you debugging and Googling skills and dive in. Good luck!